Google+ released an amazing feature that uses object recognition to tag photos.
Here is a Reddit thread discussing it. Generalized object recognition is an incredibly difficult problem - this is the first product that I have seen which supports it. This isn't a gimmick - it can recognize objects in pictures without *any* corresponding text information (such as in the filename, title, comments, etc). Here are some examples on my photos (none of these photos contain any corresponding text data to help):

At first I thought this last one was a misclassification, until I zoomed in further and saw a tiny plane:

Of course, there are also many misclassifications since this is such a hard problem:
This squirrel came up for [cat]:

This train came up for [car]:

This goat came up for [dog]:
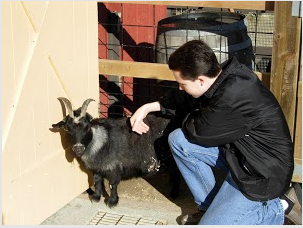
These fireworks came up for [flower]:

This millipede came up for [snake]: